Intro: This is part four of an eight part series looking at the Elevator Explorer, a fun data interactive mostly coded between the hours of 10 PM to 2 AM during the week leading up to April Fools’ Day, 2013. I’m going to be looking at the things I learned, things I wish I could have done, and the reasoning behind my design choices. The code I’ll be referring to will be in this tagged release on github.
Hey everybody, come look at the D3
So first off, this is going to go over the sketchiest code in the project. It seemed to work well for Elevators, but I have no idea how well this would translate to other projects.
At the bottom of the landing page, there’s this chart:
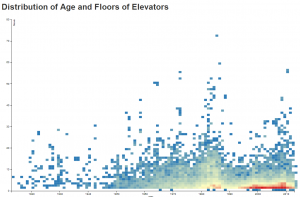
If you examine the source, you’ll see that it’s just a DIV
with data attrs that the JavaScript uses to create an IFRAME
version of the real chart full of D3 magic.
Responsive Iframes
So why use an iframe? Why not just load the chart directly into the container div? The iframe may cost us a bunch of extra HTTP requests and make it harder to integrate with the page*, but they also give us an instant embeddable. But this is the era of responsive design, and iframes are even harder to make responsive than SVGs. My solution was to insert the iframe with JavaScript. I could have just operated directly on the IFRAME
, but adding another layer (hooray more complexity) also adds the ability to serve different content based on feature detection (noscript, no svg, mobile) in the future. Why didn’t I use a preexisting library like NPR’s responsiveiframe? They were all too complicated to use (e.g. I couldn’t get them to work in 5 minutes) and I didn’t have to worry about same origin issues so I could write simpler JavaScript:
With this technique, adding an interactive visualization is as easy as adding an image. How it works is you put markup into the page similar to how you would do a VIDEO
tag:
<section>  <h2>Distribution of Age and Floors of Elevators</h2>  <div data-src="/chart/elevatorlist/">    <noscript>      Missing Figure    </noscript>  </div> </section>
And the JavaScript knows how to interpret that to insert the chart.
*Integration problems with iframes:
- Tooltips can’t extend outside the bounds of the iframe.
- Interactivity between the chart and other elements becomes difficult.
One chart, one view, one queryset
Doing iframes for charts also solves another headache: managing all the querysets that power each visualization. Powering the visualizations off a generic api is an admirable goal, but falls flat in reality. And mixing in all the querysets you need into your views makes for some massive views and maintenance headaches. Writing simple single-purpose views keeps the payload small and the code neat. You may end up with a maintenance problem of another kind, but I would rather have 10 simple small views than one unintelligible mammoth view. You can see what I ended up doing here:
The same basic Django TemplateView
handles both the chart HTML and the data needed for the visualization. Another feature is the ajax endpoint for the chart is always at the same relative URL, so for the visualization at: /chart/elevatorlist/, the data lives at /chart/elevatorlist/data.json. You don’t have to worry about moving information about your Django urlconf into JavaScript. And I’m foreshadowing here, but it’s at ./data.json and not ./data/ because it’s friendlier for wget. Another tip is to make sure you’re gzipping your json, especially for development. In my urls.py, you can see I’m using the gzip_page
decorator. But you can also enable GZipMiddleware
. This makes it much easier to know right away if your json is too large. For production, you should think about turning off gzipping in the application server and moving it up the stack.
Next steps
Like I said, this code was the sketchiest. There are many improvements to be made. Some big ones I can think of include:
- Making the chart loader JS capable of loading the chart directly into the container div instead of in an iframe.
- Adding a URL pattern helper for automatically setting up the routes for the chart template and data in one line.
- Making the API return an array of arrays instead of an array of objects to save bandwidth. It’s a little easier to throw into D3 this way too.
Live demo
Conclusion
So the title was a lie. I didn’t go into the D3 at all. Gotcha. If you’re curious, here it is. Next time, I’ll show you how a rarely used database from 1986 helped me geocode.